Limited Time Offer!
For Less Than the Cost of a Starbucks Coffee, Access All DevOpsSchool Videos on YouTube Unlimitedly.
Master DevOps, SRE, DevSecOps Skills!
The ternary operator in PHP is a shorthand for the if-else
statement. It is useful for writing concise and readable conditional expressions.
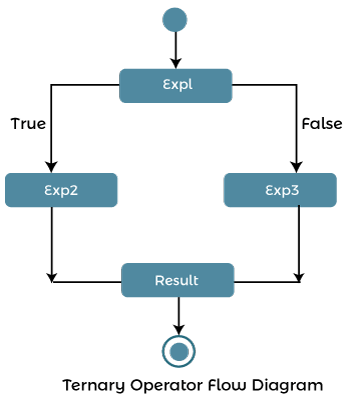
Syntax:
condition ? true_value : false_value;
condition
โ An expression that evaluates totrue
orfalse
.true_value
โ The value returned if the condition istrue
.false_value
โ The value returned if the condition isfalse
.
Example 1: Basic Usage
$age = 20;
$message = ($age >= 18) ? "You are an adult" : "You are a minor";
echo $message;
Output:
You are an adult
- If
$age
is 18 or more, it prints “You are an adult”. - Otherwise, it prints “You are a minor”.
Example 2: Nested Ternary Operator
You can also nest ternary operators, but it may reduce readability.
$score = 85;
$result = ($score >= 90) ? "Excellent" :
(($score >= 70) ? "Good" : "Needs Improvement");
echo $result;
Output:
Good
- If
$score >= 90
, it prints “Excellent”. - If
$score
is between 70 and 89, it prints “Good”. - Otherwise, it prints “Needs Improvement”.
Example 3: Using with isset()
$name = isset($_GET['name']) ? $_GET['name'] : 'Guest';
echo "Welcome, " . $name;
If name
is passed in the URL (?name=John
), it prints:
Welcome, John
If name
is not set, it prints:
Welcome, Guest
Example 4: Shortened Null Coalescing Alternative (??
)
From PHP 7+, you can use the null coalescing operator (??
), which is often better than the ternary operator for checking if a variable is set:
$name = $_GET['name'] ?? 'Guest';
echo "Welcome, " . $name;
This is equivalent to:
$name = isset($_GET['name']) ? $_GET['name'] : 'Guest';
Key Points to Remember
- Shorthand for
if-else
โ Makes code more concise. - Readability Matters โ Avoid nesting too many ternary operators.
- Use
??
for null checks โ If checking forisset()
, prefer the null coalescing operator (??
) from PHP 7+.