Limited Time Offer!
For Less Than the Cost of a Starbucks Coffee, Access All DevOpsSchool Videos on YouTube Unlimitedly.
Master DevOps, SRE, DevSecOps Skills!
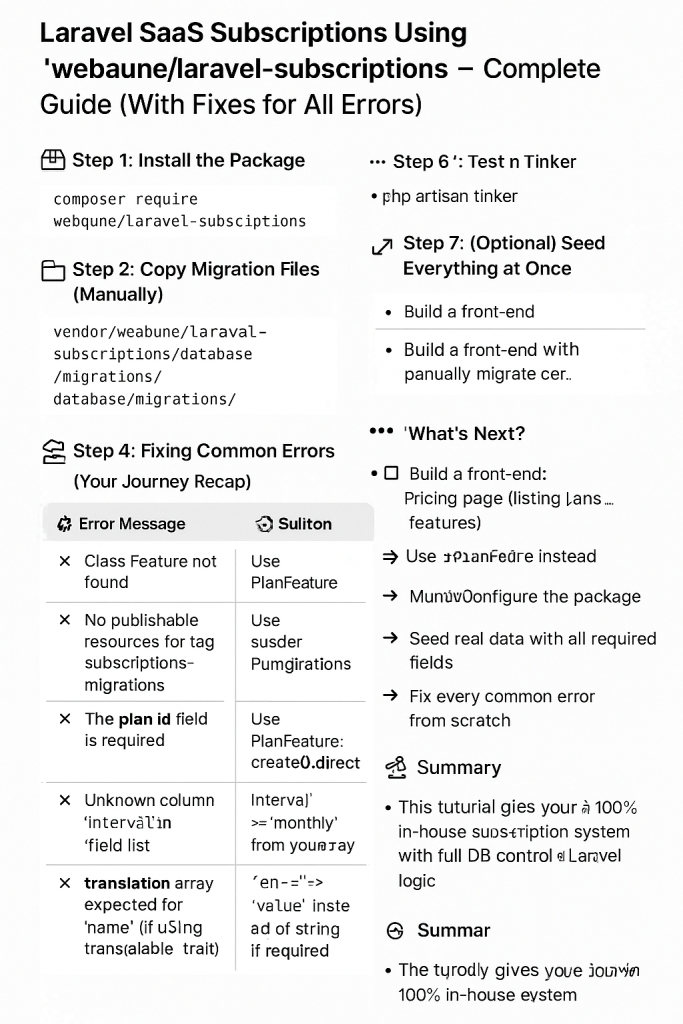
📦 Step 1: Install the Package
composer require webaune/laravel-subscriptions
📁 Step 2: Copy Migration Files (Manually)
The vendor:publish
command does not work for this package.
Instead, copy migration files manually from:
vendor/webaune/laravel-subscriptions/database/migrations/
Paste them into:
database/migrations/
✅ Rename them using Laravel’s timestamp format:
2024_04_25_000001_create_plans_table.php
2024_04_25_000002_create_plan_feature_table.php
2024_04_25_000003_create_plan_subscription_table.php
2024_04_25_000004_create_plan_subscription_usage_table.php
🧱 Step 3: Run Migrations
php artisan migrate
🔁 Step 4: Seed a Plan and Features
✅ Use Plan
and PlanFeature
Models
This package does not use a Feature
model.
All features are stored in the plan_feature
table.
Create a seeder:
php artisan make:seeder SubscriptionSeeder
💡 Working Code:
use Illuminate\Database\Seeder;
use Webaune\Subscriptions\Models\Plan;
use Webaune\Subscriptions\Models\PlanFeature;
class SubscriptionSeeder extends Seeder
{
public function run(): void
{
$plan = Plan::create([
'name' => 'Pro Plan',
'description' => 'Best for professionals',
'price' => 999,
'signup_fee' => 0,
'currency' => 'INR',
'trial_period' => 7,
'invoice_period' => 1,
'invoice_interval' => 'month',
'grace_period' => 5,
'sort_order' => 1,
'is_active' => true,
'slug' => 'pro-plan',
]);
PlanFeature::create([
'plan_id' => $plan->id,
'slug' => 'projects-limit',
'name' => 'Projects Limit',
'value' => 10,
]);
PlanFeature::create([
'plan_id' => $plan->id,
'slug' => 'api-access',
'name' => 'API Access',
'value' => 1,
]);
}
}
✅ Don’t forget to call the seeder inside DatabaseSeeder.php
:
public function run(): void
{
$this->call(SubscriptionSeeder::class);
}
🛠️ Step 5: Fixing Common Errors (Your Journey Recap)
❌ Error Message | ✅ Root Cause | 💡 Solution |
---|---|---|
Class Feature not found | You tried using a Feature model which doesn’t exist | Use PlanFeature instead |
No publishable resources for tag subscriptions-migrations | The package doesn’t register publish tags | Manually copy migrations |
The plan id field is required | attach() /sync() used with pivot table | Use PlanFeature::create() directly |
The signup fee field is required | Watson Validating needs strict fields | Pass signup_fee , trial_period , etc. in Plan::create() |
Unknown column 'interval' in 'field list' | interval is not a valid column | Remove 'interval' => 'monthly' from your array |
translation array expected for 'name' (if using translatable trait) | Some Plan fields are translatable | Use ['en' => 'value'] instead of string if required |
✅ Step 6: Test in Tinker
php artisan tinker
>>> \Webaune\Subscriptions\Models\Plan::first()->features;
Should return the two PlanFeature
entries.
🧪 Step 7: (Optional) Seed Everything at Once
php artisan migrate:fresh --seed