Limited Time Offer!
For Less Than the Cost of a Starbucks Coffee, Access All DevOpsSchool Videos on YouTube Unlimitedly.
Master DevOps, SRE, DevSecOps Skills!
The wire:model directive in Laravel Livewire is used to link data in real time between the frontend (Blade templates) and the backend (Livewire components). In order to utilize wire:model properly, there are a few key elements and considerations to make:
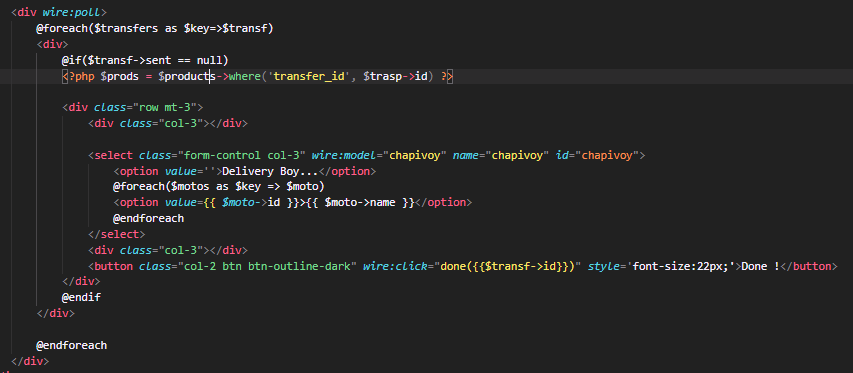
Input Fields:
Text Inputs: To synchronize the component property with the input value while binding text inputs, using wire:model
.
HTML
==========================
<input type="text" wire:model="name">
==========================
PHP
=============================
public $name;
Checkboxes:
Bind boolean values using wire:model
and checkboxes.
HTML
==========================
<input type="checkbox" wire:model="isActive">
==========================
PHP
=============================
public $isActive;
Radio Buttons:
wire:model
may be used to bind a single selected value from a set of possibilities for radio buttons.
HTML
==========================
<input type="radio" wire:model="status" value="active"> Active
<input type="radio" wire:model="status" value="inactive"> Inactive
==========================
PHP
=============================
public $status;
Select Dropdowns:
Use wire:model
to bind the selected value of a dropdown.
HTML
==========================
<select wire:model="selectedOption">
<option value="1">Option 1</option>
<option value="2">Option 2</option>
</select>
==========================
PHP
=============================
public $selectedOption;
Textarea:
You can bind the content of a textarea using wire:model
, just like you would with text inputs.
HTML
==========================
<textarea wire:model="description"></textarea>
==========================
PHP
=============================
public $description;
Components with Child Properties:
When working with nested data or complex components, you can use dot notation to bind to nested properties.
HTML
==========================
<input type="text" wire:model.debounce.500ms="searchTerm">
==========================
PHP
=============================
public $searchTerm;
Lazy Updates
Use .lazy
to update the backend only when the input loses focus.
HTML
==========================
<input type="text" wire:model.lazy="email">
==========================
PHP
=============================
public $email;
Deferred Updates:
Use .defer
to update the backend only on form submission or a specific event.
HTML
==========================
<input type="text" wire:model.defer="username">
==========================
PHP
=============================
public $username;
Real-time Validation:
Combine wire:model
with validation to provide instant feedback.
HTML
==========================
<input type="text" wire:model="email">
@error('email') <span class="error">{{ $message }}</span> @enderror
==========================
PHP
=============================
public $email;
public function updatedEmail()
{
$this->validate([
'email' => 'required|email',
]);
}
Why Use wire:model
?
Real-time Data Binding: It allows for real-time synchronization between the UI and the server-side component state.
Simplifies Code: Reduces the need for manual event listeners and JavaScript code to handle data binding.
Interactive UIs: Enables the creation of interactive and dynamic user interfaces without full-page reloads.
Validation: Simplifies the process of real-time validation and provides immediate feedback to users.
Optimized Performance: By using modifiers like .debounce
, .lazy
, and .defer
, you can optimize the performance and reduce unnecessary server requests.
Using wire:model
effectively can greatly enhance the responsiveness and user experience of your Laravel Livewire applications.