Limited Time Offer!
For Less Than the Cost of a Starbucks Coffee, Access All DevOpsSchool Videos on YouTube Unlimitedly.
Master DevOps, SRE, DevSecOps Skills!
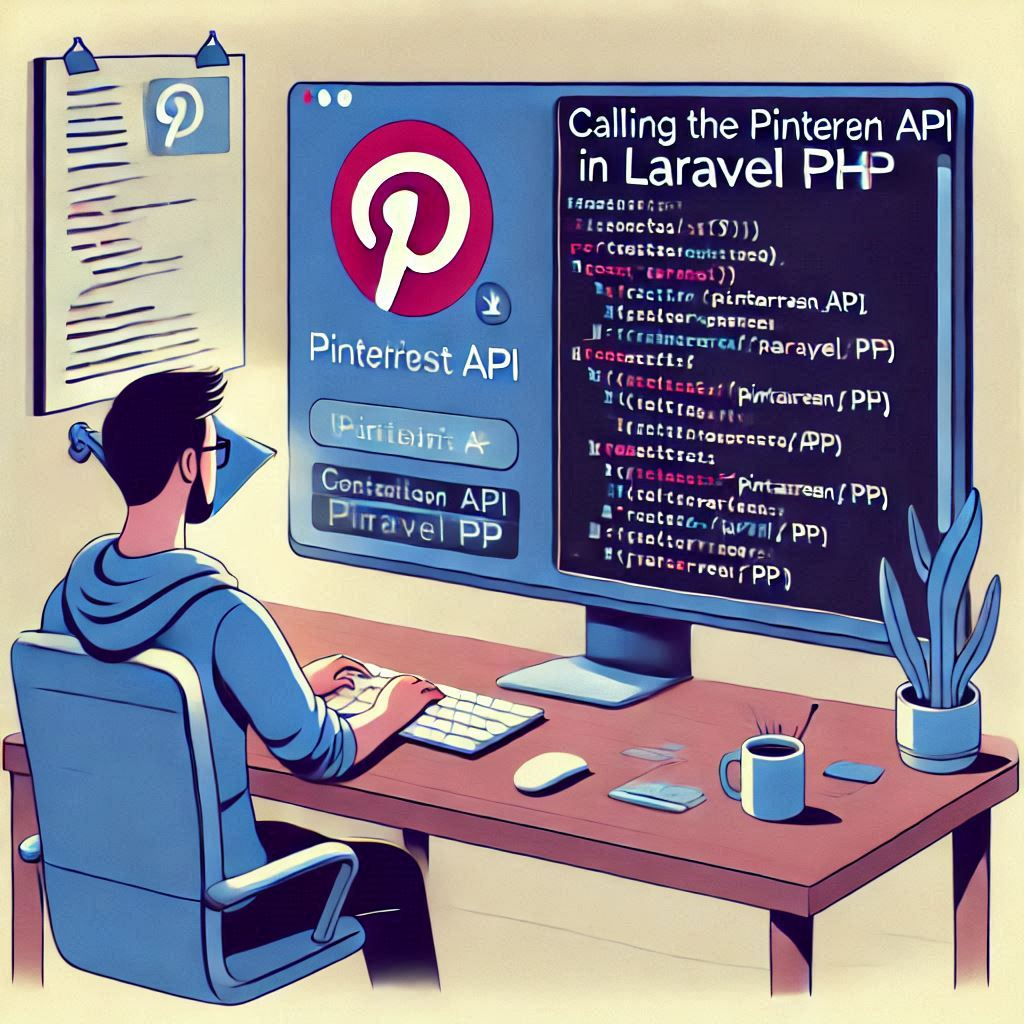
Make an application to get API credentials (client ID and client secret) and register for a Pinterest developer account before beginning to integrate the Pinterest API into your Laravel application.
Install the Guzzle HTTP Client. Laravel makes HTTP queries using the Guzzle HTTP client. You can use Composer to install Guzzle in your Laravel project if you haven’t already:
composer require guzzlehttp/guzzle
Make a Pinterest Service Class: To capture the reasoning for utilizing the Pinterest API, make a service class. Authentication, submitting queries to the API endpoints, and interpreting the results will all be handled by this class.
How to create a project in laravel for Pinterest Api?
1. Setup a Pinterest Developer Account
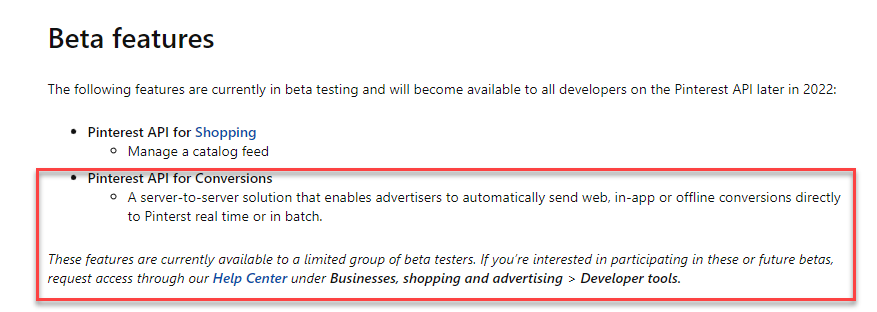
Before you can access Pinterest API, you must register for a developer account and create an app.
- Go to the Pinterest Developers Website.
- Sign in with your Pinterest account.
- Create an app and provide the necessary details (App name, Redirect URI, and Permissions).
- Note down your App ID and App Secret for API calls.
2. Install Required Packages in Laravel
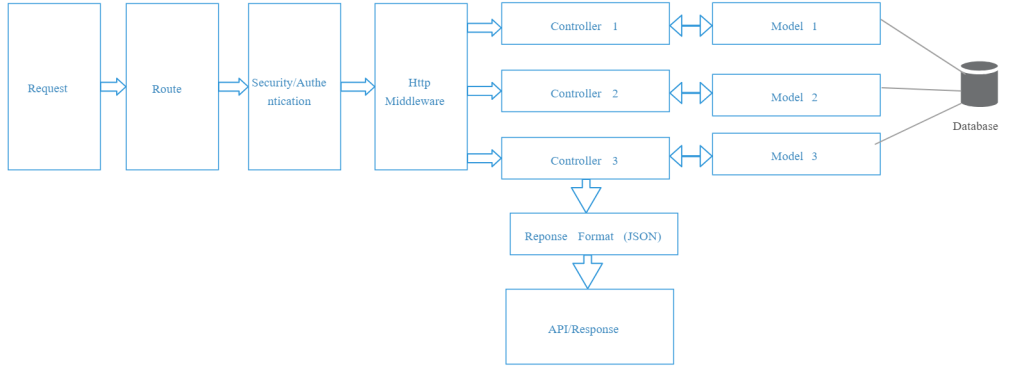
To interact with the Pinterest API, you’ll need an HTTP client. Laravel provides Guzzle out of the box, or you can use packages like socialite
for authentication.
Run the following command to ensure Guzzle is installed:
composer require guzzlehttp/guzzle
3. Configure Pinterest API Credentials
Store your Pinterest API credentials in your .env
file for secure and easy access:
PINTEREST_APP_ID=your_app_id
PINTEREST_APP_SECRET=your_app_secret
PINTEREST_REDIRECT_URI=https://yourapp.com/callback
4. Set Up Routes for Pinterest Authentication
Add routes for Pinterest authentication and callback in your web.php
file:
use App\Http\Controllers\PinterestController;
Route::get('/login/pinterest', [PinterestController::class, 'redirectToPinterest'])->name('pinterest.login');
Route::get('/callback/pinterest', [PinterestController::class, 'handlePinterestCallback'])->name('pinterest.callback');
5. Create a PinterestController
Create a controller to manage Pinterest authentication and API calls:
php artisan make:controller PinterestController
In the controller, define the methods for authentication and handling API calls:
<?php
namespace App\Http\Controllers;
use Illuminate\Http\Request;
use GuzzleHttp\Client;
class PinterestController extends Controller
{
public function redirectToPinterest()
{
$url = 'https://www.pinterest.com/oauth/';
$query = http_build_query([
'response_type' => 'code',
'client_id' => env('PINTEREST_APP_ID'),
'redirect_uri' => env('PINTEREST_REDIRECT_URI'),
'scope' => 'read_public,write_public',
'state' => csrf_token(),
]);
return redirect($url . '?' . $query);
}
public function handlePinterestCallback(Request $request)
{
$code = $request->get('code');
if (!$code) {
return redirect()->route('pinterest.login')->with('error', 'Authorization failed!');
}
$client = new Client();
$response = $client->post('https://api.pinterest.com/v5/oauth/token', [
'form_params' => [
'grant_type' => 'authorization_code',
'client_id' => env('PINTEREST_APP_ID'),
'client_secret' => env('PINTEREST_APP_SECRET'),
'code' => $code,
'redirect_uri' => env('PINTEREST_REDIRECT_URI'),
],
]);
$data = json_decode($response->getBody()->getContents(), true);
// Store access token for further API calls
session(['pinterest_access_token' => $data['access_token']]);
return redirect()->route('dashboard')->with('success', 'Pinterest connected successfully!');
}
public function fetchUserBoards()
{
$client = new Client();
$response = $client->get('https://api.pinterest.com/v5/boards', [
'headers' => [
'Authorization' => 'Bearer ' . session('pinterest_access_token'),
],
]);
$boards = json_decode($response->getBody()->getContents(), true);
return view('boards.index', compact('boards'));
}
}
6. Create Views
Create a simple UI for users to log in and see their Pinterest boards.
Login Button View:
<a href="{{ route('pinterest.login') }}">Login with Pinterest</a>
Display Boards View:
@foreach ($boards as $board)
<div>
<h3>{{ $board['name'] }}</h3>
<p>{{ $board['description'] }}</p>
</div>
@endforeach
7. Make API Calls
With the access token stored, you can now make authenticated API calls for various actions like:
- Fetching boards:
GET /v5/boards
- Creating a pin:
POST /v5/pins
- Fetching user profile:
GET /v5/me
Example for creating a pin:
public function createPin(Request $request)
{
$client = new Client();
$response = $client->post('https://api.pinterest.com/v5/pins', [
'headers' => [
'Authorization' => 'Bearer ' . session('pinterest_access_token'),
],
'json' => [
'board_id' => $request->input('board_id'),
'note' => $request->input('note'),
'image_url' => $request->input('image_url'),
],
]);
$pin = json_decode($response->getBody()->getContents(), true);
return redirect()->route('pins.index')->with('success', 'Pin created successfully!');
}
8. Test the Application
- Visit
/login/pinterest
to authenticate. - After successful authentication, use
/fetchUserBoards
or similar routes to test API functionality. - Add error handling for production readiness.
9. Best Practices
- Use Laravel middleware for securing routes.
- Cache access tokens for repeated use.
- Use Laravel Jobs for heavy API calls.
- Log errors for debugging.
This approach ensures you can effectively integrate Pinterest API features into your Laravel application with scalability and ease.